안녕하세요~ 효그니에여 ><
오늘 날씨가 뭔가 꿀꿀해서 돼지고기가 먹고싶어지네요(?)
뭐 어쨌든 각설하고!
오늘은 ListView란 뷰를 배워볼거에요!
ListView는 스크롤 가능한 항목을 나타낼 때 사용되는 뷰 그룹이에요!
ListView는 먼저 View를 배치한 다음, 데이터가 저장된 곳에서 데이터를 형식에 맞춰 가져와요!
그럼 개념은 대충 설명 했으니 바로 안스를 켜볼까요?
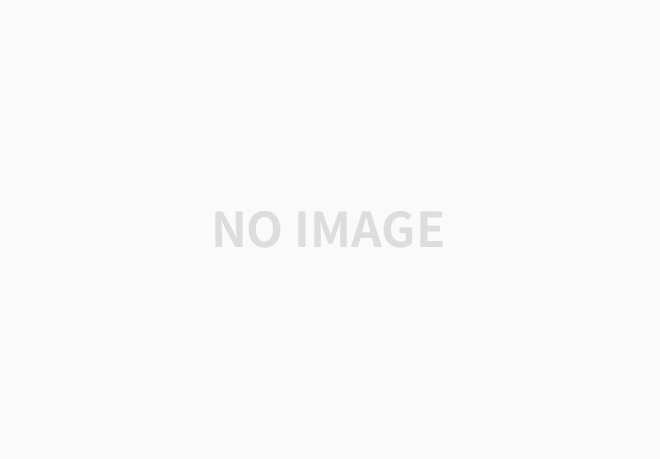
<ListView
android:id="@+id/list_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent">
</ListView>
이렇게 일단 리스트뷰를 만들어줘요!
그 다음에는 User.kt파일을 생성해줘요!
리스트뷰를 사용하기 위해서는
- Data Class
- XML에 ListView 추가
- item 생성
- Adapter 생성
- Adapter 설정
의 총 5가지의 과정이 필요해요!
이번엔 유저 목록을 만들것이기에 User.kt라는 Data Class를 정의해주는거에요! 그럼 생성해 볼까요?
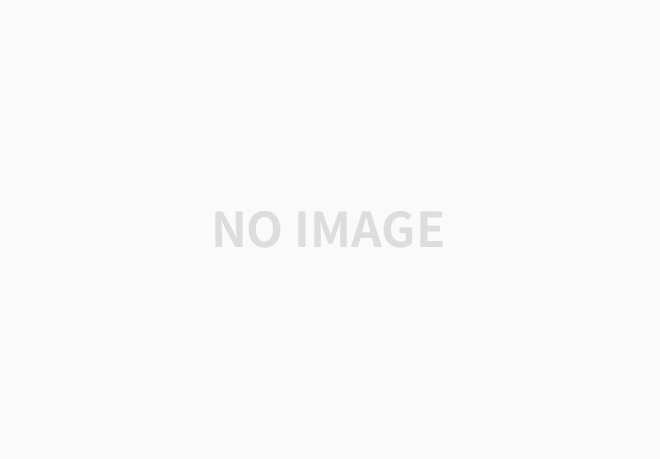
class User (val name: String, val email: String, val content: String)
이렇게요!
그리고 Item을 생성해볼까요?
layout에 item_user이라는 파일을 생성해줘요!
여기에서 등장하는게 CardView에요!
CardView 는 support.v7.widget 에 속한 라이브러리에요!
CardView는 FrameLayout 클래스를 확장한 형태라고 보시면 되요!
이 CardView를 왜 사용하냐구요?
당연한거죠! "이뻐서" ㅎㅎ... 가독성도 좋구여 ㅎㅎ...
각설하고,
CardView를 사용하기 위해서는 일단 Build.Gradle에
implementation 'com.android.support:cardview-v7:28.0.0'
을 추가 해줘야해요!
그리고 다시 item_user.xml에 넘어와서
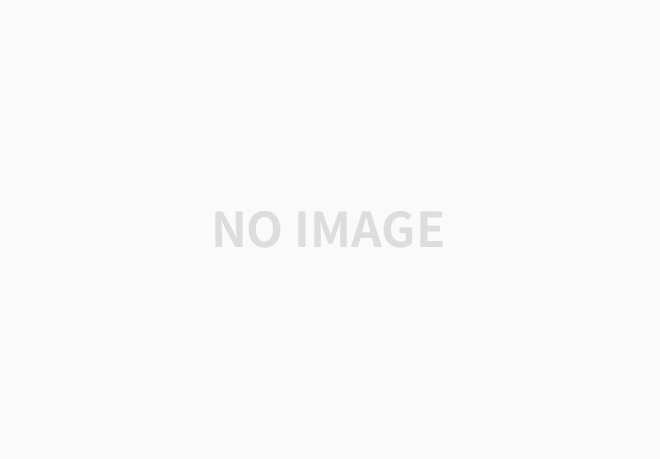
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:card_view="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:id="@+id/cardview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="18.3dp"
android:layout_marginEnd="18.7dp"
android:layout_marginTop="11dp"
card_view:cardCornerRadius="17dp"
android:layout_margin="10dp">
<RelativeLayout
android:id="@+id/firstLin"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="#ffffff"
android:padding="10dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/imageIcon"
android:layout_width="52dp"
android:layout_height="52dp"
android:layout_margin="10dp"
android:layout_marginEnd="10dp"
android:src="@mipmap/ic_launcher" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="10dp"
android:layout_toRightOf="@+id/imageIcon"
android:orientation="vertical">
<TextView
android:id="@+id/name_tv"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="14sp"
android:text="가나다" />
<TextView
android:id="@+id/email_tv"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="12sp"
android:text="마바사"/>
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="15dp"
android:layout_below="@+id/imageIcon">
<TextView
android:id="@+id/content_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="가나다라"
android:textSize="15sp"/>
</LinearLayout>
</RelativeLayout>
</RelativeLayout>
</android.support.v7.widget.CardView>
</LinearLayout>
이렇게 짜주시면 되요!
그 후 Adapter를 만들어주셔야 하는데
ListView의 각 item, 그리고 거기에 넣을 데이터까지 설정했으면 Adapter를 만들어야해요.
어느 요소를 어느 View에 넣을 것인지 연결해주는 것이 Adpater의 역할이에요!
ListAdapter라는 파일을 생성해줘요!
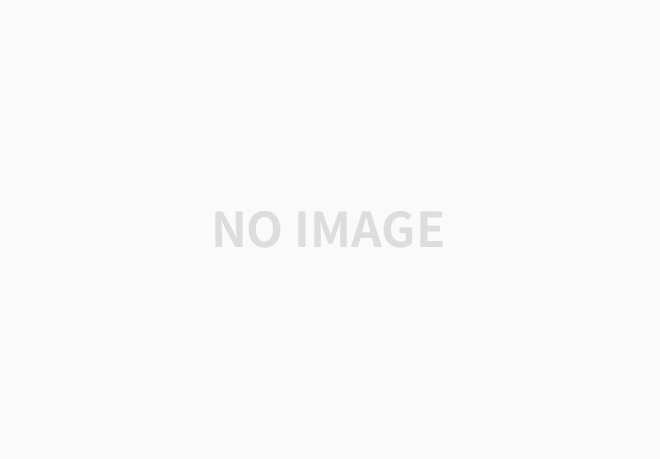
이렇게요!
class ListAdapter (val context: Context, val UserList: ArrayList<User>) : BaseAdapter() {
override fun getView(position: Int, convertView: View?, parent: ViewGroup?): View {
val view: View = LayoutInflater.from(context).inflate(R.layout.item_user, null)
val Name = view.findViewById<TextView>(R.id.name_tv)
val Email = view.findViewById<TextView>(R.id.email_tv)
val Content = view.findViewById<TextView>(R.id.content_tv)
val user = UserList[position]
Name.text = user.name
Email.text = user.email
Content.text = user.content
return view
}
override fun getItem(position: Int): Any {
return UserList[position]
}
override fun getItemId(position: Int): Long {
return 0
}
override fun getCount(): Int {
return UserList.size
}
}
이렇게 생성해주신 후 Adapter를 설정해주셔야 하는데 MainActivity.kt로 돌아오셔서
var UserList = arrayListOf<User>()
처럼 초기화 시켜주셔야해요
그 후 ListView와 Adapter를 연결해주셔야해요!
var UserList = arrayListOf<User>()
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val Adapter = ListAdapter(this, UserList)
list_view.adapter = Adapter
}
이렇게요!
그리고 실제로는...서버랑 연동을 해서 데이터를 받아와야하겠지만 아직 Retrofit Service를 배우지 않았으니!
하드코딩 합시다 핳하하하하핳.....
var UserList = arrayListOf<User>(
User("심효근","shimhg02@naver.com","아령하세요잇!"),
User("박채연","asdf@naver.com","할말이 없다"),
User("박서연","qwerqr2@naver.com","ㄷ"),
User("박태욱","ㅁㄴㅇㄹㅁㄴㅇㄹㅁㄴㅇㄹ@naver.com","ㅁㄴㅇㄹ"),
User("김민식","qwer2@naver.com","ㅇㅁㄴㄹ!"),
User("이소명","shㅇㄹ@naver.com","아령dsafsdf!"),
User("한규언","shiㅁㄴㅇㄹ@naver.com","afsdf!"),
User("정빈","shi@naver.com","ㅁㄴㅇㄹ"),
User("김태양","sㅁㄴㅇㄹㅁㅇㄴㄹaver.com","아ㅇ잇!")
)
이렇게 말이죠. (즉석으로 떠오르는 이름 아무거나 쓴거에요 ㅎㅎ,,,)
그리고 실행해볼까요?
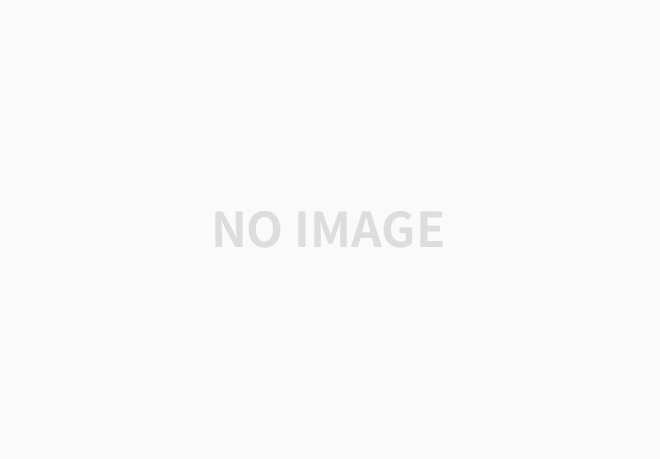
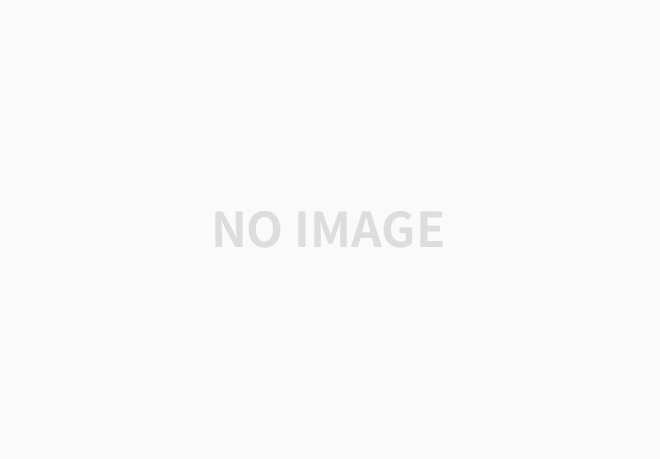
정상적으로 실행이 되는군요!!
오늘 배워본건 Android ListView에요!
후후 오늘도 열심히 공부하는 당신에게 박수를!!!
오늘 하루도 좋은 하루 되세요~!
'Android > Android Lecture' 카테고리의 다른 글
안드로이드 강의 9. TabLayout & ViewPager 와 BaseActivity사용 (0) | 2019.04.10 |
---|---|
안드로이드 강의 8. Fragment를 사용하자! (4) | 2019.04.09 |
안드로이드 강의 6. Handler를 이용한 Splash화면 구현 (2) | 2019.04.08 |
안드로이드 강의 5. Intent와 Activity 이동 (5) | 2019.04.08 |
안드로이드 강의 4. Button, OnClickListener, Toast 메시지 (1) | 2019.04.08 |